Recently I worked on a proof of concept with a requirement of language translation in a Web application. There are a lot of Language translation services available in the market but most of them failed with more than one parameters I checked for. The parameters I used for this study are Quality, Performance, Pricing, Ease of integration, Languages supported. As part of the exercise, I tried integrating some of the leading translation services with the .NET application. This is the first post in the series and i am going to talk about the integration of Google Translation API with Dotnet Core in this.
Google Translation API helps translate text from one language to another instantly. It uses Google’s pre-trained neural machine translation to do the translation. Also, the API supports multiple technologies to integrate with the translation service programmatically. Let’s start with the benefits of the Google Translation API.
In this post, we will discuss the following
- Benefits of Google Translation API
- Enable the service in Google Cloud Platform Console
- Integrate Google Translation API in ASP.NET Core MVC Application
- Pricing
Benefits
- Translate more than one hundred languages as of this writing
- Automatic language detection
- Easy integration supports multiple technologies (C#, Python, Ruby, Node.js, etc.)
- Affordable – Per character basis(refer to the “Pricing” section)
The integration of Google Translate API with ASP.NET Core application involves enabling the Google Translate API and create an ASP.NET Core Web Application. I am going to talk about three use cases in this post. The first one is translating text directly in the MVC Controller and return it. The language is hard-coded here. The second use case is about letting the user selects a language and let them translate it. I have used jQuery and Web API Controller for this. The last one is similar to the second, but it translates HTML instead of plain text.
Prerequisites
- A Google Account
- .NET Core 2.2 SDK
- VS Code, Visual Studio 2017/2019
- Familiarity with ASP.NET MVC, jQuery
Enable the Google Translation API
You need a paid account to create a Google Translate API Key. Though Google asks to set up the payment details, they provide $300 credit which is valid for a year to access the Google Cloud Platform.
Go to the Cloud Platform Console
Create a new project
Click on “Enable APIS and Services” to see the list of services
Search for “translate”, select “Cloud Translation API” and activate it
Create a service account and download a private key as JSON
Getting started with ASP.NET MVC application
Once you created the application, set the environment variable with the key “GOOGLE_APPLICATION_CREDENTIALS” to the file path of the Service account key JSON file you have downloaded earlier.
Install “Google.Cloud.Translation.V2” Nuget package in the solution
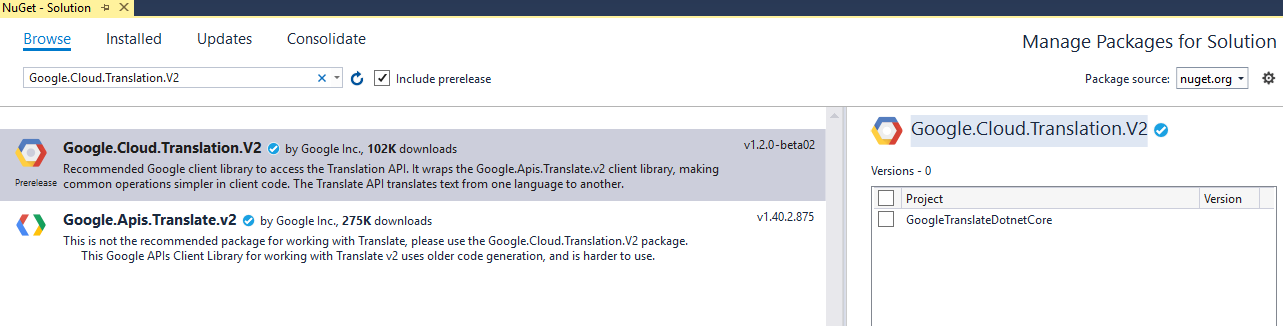
The following code handles the actual translation by calling the Google Translation API. I have accessed this from the controllers. I have configured the “Translator” class as a singleton in the startup class.
Translator.cs
using Google.Cloud.Translation.V2; namespace GoogleTranslateDotnetCore.Utils { public interface ITranslator { string TranslateText(string text, string language); string TranslateHtml(string text, string language); } public class Translator : ITranslator { private readonly TranslationClient client; public Translator() { client = TranslationClient.Create(); } public string TranslateHtml(string text, string language) { var response = client.TranslateHtml(text, language); return response.TranslatedText; } public string TranslateText(string text, string language) { var response = client.TranslateText(text, language); return response.TranslatedText; } } }
I have called the translation code from the controller. The source text and language are hard-coded here. You can get the source and the language from model or viewdata.
HomeController.cs
using System.Diagnostics; using Microsoft.AspNetCore.Mvc; using GoogleTranslateDotnetCore.Models; using GoogleTranslateDotnetCore.Utils; namespace GoogleTranslateDotnetCore.Controllers { public class HomeController : Controller { private readonly ITranslator _translator; public HomeController(ITranslator translator) { _translator = translator; } public IActionResult Index() { var text = "Translate using Google"; var translatedText = _translator.TranslateText(text, "fr"); return View("Index", translatedText); } } }
Translate text and HTML by getting user input
I have created an API Controller and calling it using jQuery. The API Controller calls the “Translator” and do the translation. You can see two translate methods here. The “Translate” method takes care of text translation while the “TranslateHtml” method helps with HTML translation.
using GoogleTranslateDotnetCore.Utils; using Microsoft.AspNetCore.Mvc; namespace GoogleTranslateDotnetCore.Controllers { [Route("api/[controller]")] [ApiController] public class TranslatorController : ControllerBase { private readonly ITranslator _translator; public TranslatorController(ITranslator translator) { _translator = translator; } [HttpGet] [Route("Translate")] public ActionResult Translate(string text, string language) { var translatedText = _translator.TranslateText(text, language); return Ok(translatedText); } [HttpGet] [Route("TranslateHtml")] public ActionResult TranslateHtml(string html, string language) { var translatedText = _translator.TranslateHtml(html, language); return Ok(translatedText); } } }
Following is the view which calls the API Controller using jQuery. Translation happens when the user selects a language from the dropdown. I haven’t done any validations to check the source and the selection.
Translator.cshtml
@{ ViewData["Title"] = "Translator"; Layout = "~/Views/Shared/_Layout.cshtml"; } <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script> <div class="text-center"> <h1 class="display-4">Welcome</h1> <p>Learn how to use Google Translate API in Dotnet Core</p> </div> <div class="container"> <div class="row"> <div class="col-sm"> <b>Source:</b> <br /> <textarea id="source" rows="10" cols="50"></textarea> </div> <div class="col-sm"> <b>Language</b> <br /> <select id="language"> <option value="">Select</option> <option value="en">English</option> <option value="zh">Chinese (Simplified)</option> <option value="ta">Tamil</option> <option value="fr">French</option> <option value="ru">Russian</option> </select> </div> <div class="col-sm"> <b>Target:</b> <br /> <textarea id="target" rows="10" cols="50"></textarea> </div> </div> </div> <script> $(function () { $("#language").change(function () { var url = '@Url.Action("Translate","Translator")'; $.get(url, { 'text': $('#source').val(), 'language': $(this).val() }) .done(function (data) { $('#target').val(data); }); }); }); </script>
The result page will be like this after the translation. The sample code contains another page similar to this but translates HTML. The “TranslateHtml” method does not translate any HTML tags in the input. Currently, there is no support for other markup languages like XML.
Pricing
Translation API is priced based on the usage calculated in millions of characters. It costs $20 per million character of text translated. Google does not charge extra for language detection when you do not mention the source language for the translate method. But there is a price applies for the detect method.
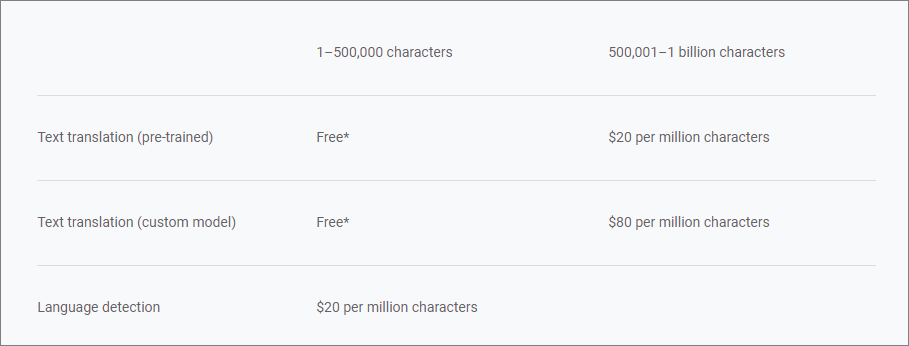
Summary
In this post, you saw how to use Google Translation API with Dotnet Core application. You learned how to use the Google Translation API to translate text on server-side using MVC Controller and on client-side using Web API Controller and jQuery.
The full implementation of the sample code used in this post can be found on GitHub.
Pingback: Using Google Translation API with Dotnet Core - Blog of Pi - How to Code .NET
Pingback: Google Translation API with Dotnet Core - How to Code .NET